9 Common ReactJs Mistakes To Avoid In 2024
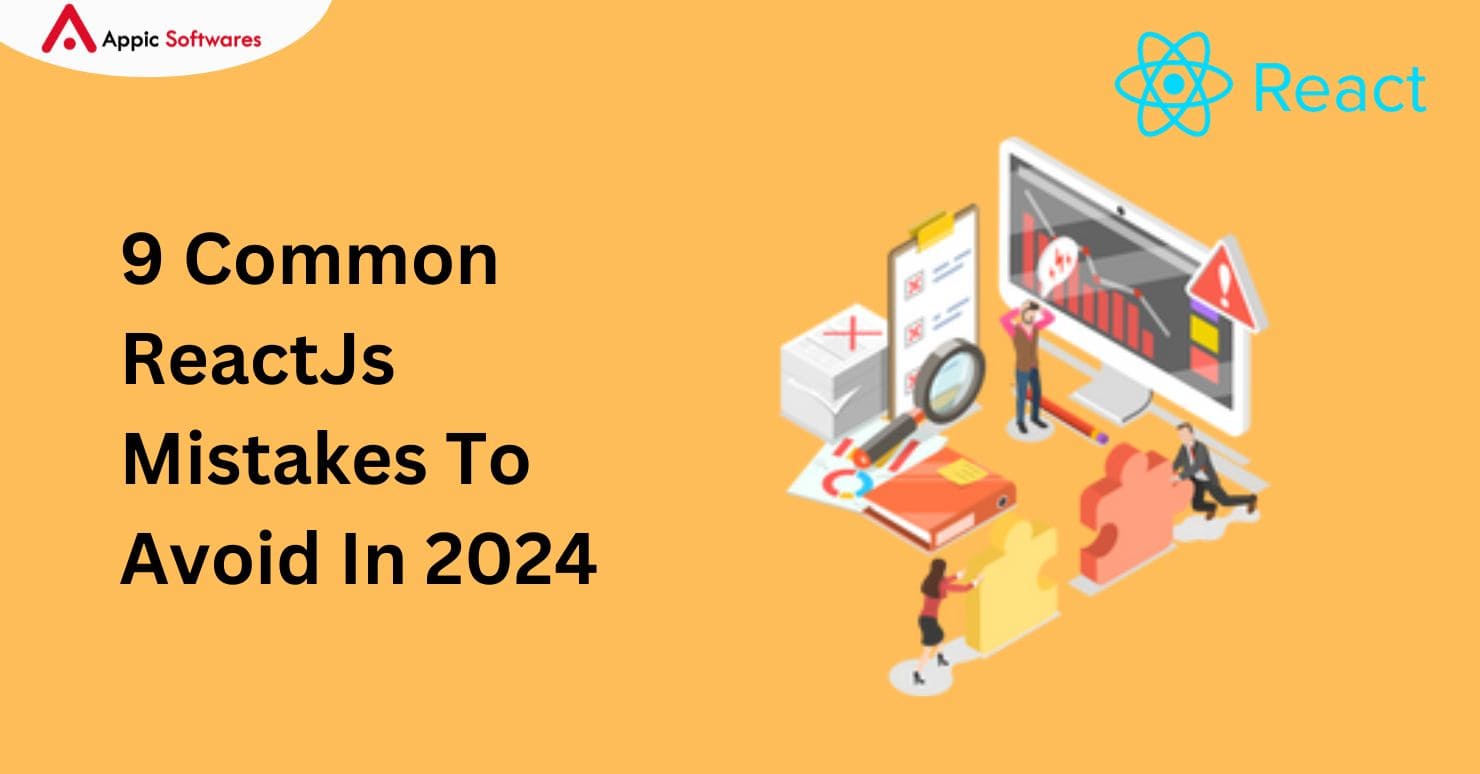
Developers all throughout the world now frequently hear the term “React.” Its creation has led to its immense popularity. React’s lack of strong opinions about how to be used is one of its best features, which can make it very effective and simple to understand. Excessive flexibility, meanwhile, can also facilitate the development of undesirable behaviors and unhealthy habits.
It is possible for practices and habits to differ among developers, teams, and companies; therefore, you may encounter whole different methods of operation when you relocate to a new setting. In the end, knowing all the different approaches to React code can help any developer identify the best one. As engineers and developers, our mission should always be to use best practices and create the greatest product possible given the limitations at hand.
These are a few of the worst things I’ve ever seen (and maybe even done myself ). With any luck, this will assist you in avoiding repeating these errors in the future.
1. Prop Drilling
Prop drilling is the technique of giving a child a single prop that is buried several levels deep by a parent.
Going more than two layers deep is ideal when transmitting a prop from a parent to a youngster. Parent -> child (layer 1) -> child (layer 2) relationships You can actually utilize as many layers as you like, but keep in mind that prop drilling can be very problematic for React codebases in terms of performance.
Specifically, prop drilling may result in needless re-rendering. Intermediate components that are only passing a prop down the chain will be compelled to render when passing the prop down the chain because React components always render when a prop is modified. Long-term performance issues with an application may result from this.
Furthermore, figuring out how data is transferred through an application is not enjoyable. Finding out how data is being used becomes more difficult for someone trying to understand an application when props are nested many levels deep.
There are numerous approaches to solving this problem. You may try rearranging your components, utilizing Redux, or the react context hook. Regrettably, for lesser apps, Redux tends to be overkill and demands a considerable bit of overhead and organization. Redux, however, might be the ideal option in situations where you need to alter several things in response to a single state change or if one state must be the result of another.
2. Importing more than you need
After all, React is a front-end framework. It is a library that builds your user interface (UI) for your users and is deployed to all browsers and mobile devices upon request. React applications should be as small and light as possible to prevent sending users more information than is necessary. Bloat and unnecessary dependencies cause an application to perform worse and take longer for users to load, giving the impression that it is slow.
Your First Contentful Paint should load quickly (preferably in the range of 0 to 1.8 seconds) to ensure that users enjoy a positive experience with your application. Therefore, minimize the amount of shipping code to simplify your bundle. The majority of contemporary app bundlers, including as Parcel and webpack, perform a great deal of the work to minify and compress your code for production; nonetheless, it’s best to be conscious of the code that’s being sent to your client and not just rely on app bundlers to try to make things right.
For instance, when lodash was developed, it had many features that JavaScript did not. Lodash is no longer relevant because important improvements have been made to current JavaScript. To evaluate if what you’re attempting to accomplish even needs lodash anymore, check out You Might Not Need Lodash. Granted, this also relies on the JavaScript version that your users are running, but most React apps have Babel or another transpiler installed to handle this for you.and now days, Chrome is the only browser that anyone supports, right?
3. Not separating business logic from component logic
In general, it’s preferable to make your components as “UI related” as you can. To handle any state that has to be displayed and present what needs to be displayed, these components should have straightforward logic and code. It’s ideal to abstract away the logic behind the API request into a separate service utility file or something similar, even though a component might need to make an API call on the initial render to retrieve the data it needs to display.
Two benefits come from separating business logic from component logic: one is able to reuse the business logic elsewhere and the other is able to keep concerns apart. A user interface component may get too complex due to the logic needed to display many UI elements. Business logic will simply serve to further complicate the element and increase the difficulty of comprehending what is happening.
4. Duplicated work on each render
Rendering can occur frequently and without warning. When creating React components, bear in mind that this is one of the fundamental principles of creating resilient components. Regretfully, this implies that any work completed during a component’s rendering will be repeated for each render. This is where React’s useMemo and usecallback hooks can be useful. These hooks can improve efficiency when used correctly by memoizing some processes that don’t need to be done again on every render.
In this example, our component is showing the user a list of objects that have been filtered based on a parameter. This is an excellent example of how to use useMemo on a list to stop it from refiltering every render and only filter when the items or the filter itself changes. This kind of filtering isn’t always avoidable on the front end.
5. Using the use effect hook improperly
Most likely, one of the first hooks a React developer learns is the useEffect hook. UseEffect has replaced componentDidMount, a popular lifecycle function used in the days of React’s class components for assigning event listeners, in the world of hooks. On the other hand, if you use the useEffect hook incorrectly, you will wind up with several event listeners.
React usage is still valid even if you remove the function return here unless you have some kind of linting set with the appropriate rules. Additionally, to guarantee that the useEffect hook will only be performed once, it’s crucial to pass the empty dependency array as the second option. The absence of the return function in a useEffect hook that sets up event listeners is all too common, and it can result in some difficult-to-debug issues.
6. Incorrect usage of Boolean operators
To render or not render specific HTML elements on the page, the majority of components have numerous Booleans in place. This is entirely typical. Nonetheless, a component can handle this logic in a few different ways. The operator “&&” is the most often used one in a component. Although this JavaScript is fully functioning, there may be some unexpected effects on your user interface. For instance:
In this case, we would like a component to display the number of products in a shopping cart; however, in this case, the website will just display “0.” Although JavaScript’s truthy/falsy comparison works when using a conditional render syntax like this, it’s preferable to use Boolean instead because it can have unexpected side consequences in React.
There are a variety of methods for handling this, and in the end, it may come down to taste. In certain cases, it may be more sensible to separate a conditionally displayed component into a separate component and just return null in the event that the condition isn’t satisfied. The most practical approach to conditionally render something, in my opinion, is to attempt converting numbers, arrays, texts, etc. into Booleans for the condition you are verifying.
7. Conditionally rendering using ternary operators everywhere
When applied properly, a classic ternary statement can be immensely fulfilling. However, using it to render options within a React component can be all too tempting. These expressions have the drawback of sometimes enveloping big components, making them difficult to understand when reading the code.
Although each part of the ternary expression contains three lines of code, it still appears crowded even if there is nothing functionally incorrect. As the code grows, it might become difficult to comprehend, especially when ternary functions or other conditional rendering options are nested inside of them. As alternatives to this ternary phrase, think about a few of these possible choices.


Because it allows you to utilize JavaScript in a more comfortable way and accomplish more intricate rendering logic, Option 1 is a favorite. Option 2 is likewise functional, but in a typical situation, the two components that are conditionally drawn would probably be in different files, and it could be more challenging to maintain consistency in the rendering logic should something change later on. Option 1 allows you to modify your rendering logic in only one location. In addition, Option 2’s reasoning is a little trickier to understand than our helper function’s.
8. Not classifying props or disassembling them
React stores prop values for components in objects, just like other JavaScript applications do. This implies that a wide range of values can be supplied within the object without the component having easy access to them. It could be difficult to work on the relevant component as a result.
There are numerous methods to tackle this. Using the above BestExample in a JavaScript context will yield two distinct advantages. You will first be aware of the exact location of your component’s definition and the props that it can access. This is advantageous because you won’t have to search far and wide for props later on if you want to alter something.
You may also learn more about what the component will anticipate in order to work correctly by utilizing propTypes on the component. You will also see warnings in the console of your web browser when specific requirements aren’t fulfilled on the props of the component. Let’s say that, rather of the usual string, the title or content in this case is provided in as undefined or a number.
9. For larger applications, do not use code splitting
Big UI bundles are indicative of huge apps. It can get rather big with all the bespoke parts and libraries that are needed. Code splitting makes it possible to effectively “split” your bundle into smaller, more manageable chunks that can be loaded and requested at a later time. This also makes the first piece of code required to operate your app more quickly available to your customer base.

Code splitting should be taken into consideration for modals, routes that a user might not visit initially, and mobile/desktop user interfaces. There are a ton of possible use cases for this, but in the end, it’s up to the product teams and developers to comprehend how users interact with the program and determine which areas are most sensible to load the app first. You can utilize the code splitting examples provided by React. Additionally, React-loadable is a functional NPM package that you may want to investigate. Some nice patterns in React-loadable also initiate a request to fetch necessary assets when an element is hovered over. This functions really well on a button that may open a split modal.
Conclusion
With the help of React, we can build user interfaces and experiences for our users more quickly and easily than ever before. Sadly, it’s only a collection of tools, and anything can be abused. We can produce more readable and useful code by utilizing tools as intended and JavaScript features in the desired way. It’s a good idea to try to improve our code as developers and make it easier for other developers to work with our code.
Moreover, if you are looking for an experienced team of ReactJs developers, you can hire them dedicatedly and let them manage your resources. Appic Softwares have a vetted team of ReactJs developers that can manage your project.
So, what are you waiting for?