Tips For Performance That Every Flutter Developer Should Know
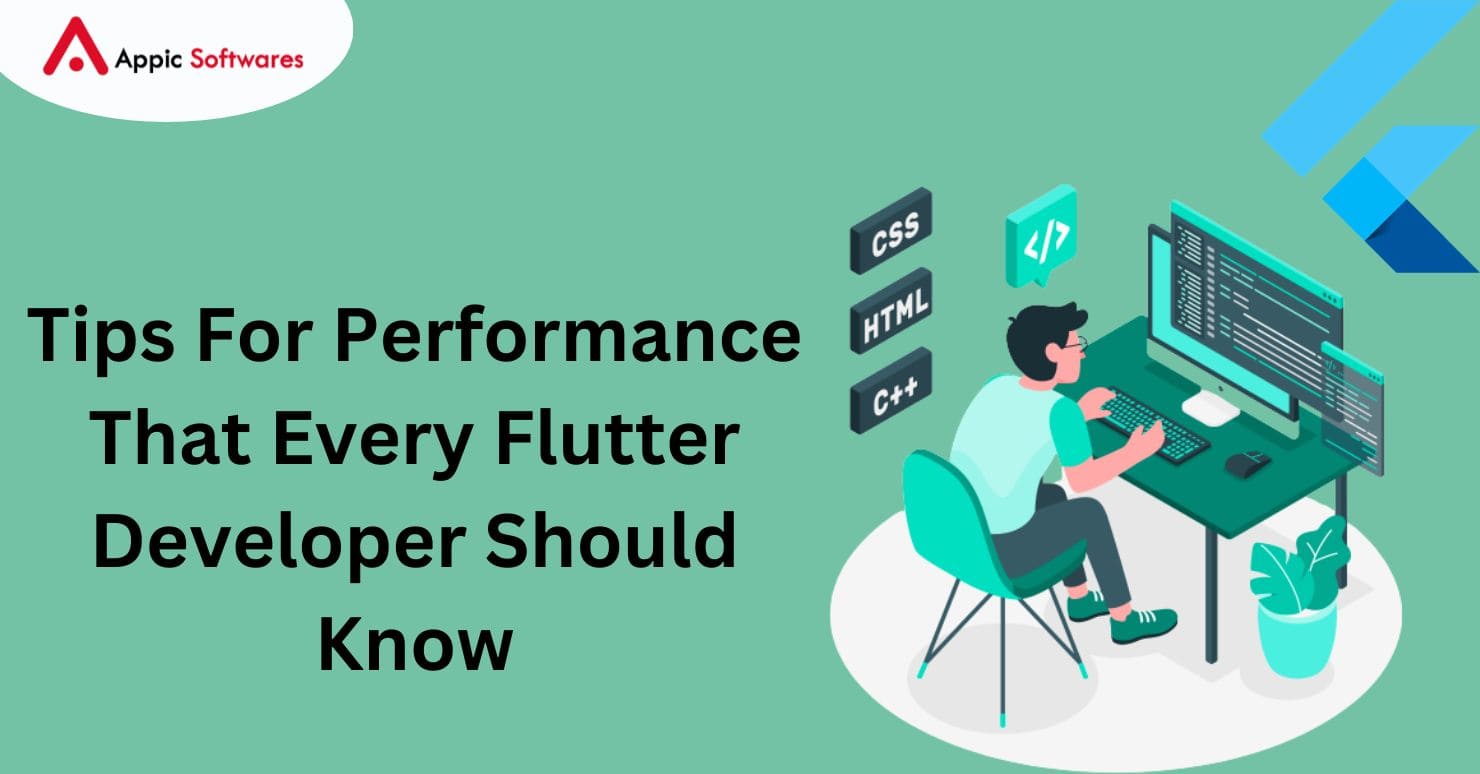
Flutter is the best cross-platform app development technology, and its popularity is growing quickly. It gives developers a lot of options for mobile app development services. As the business grows, it needs to figure out how to tell the difference between good practices and bad ones and what the best way to handle each case is.
When you’re writing, especially when making Flutter apps, performance optimization is very important. But getting the most out of your app can be hard, but it’s not impossible.
This article will talk about some of the best ways to plan and build your next project with Flutter, with a focus on making the code better, easier to read, easier to manage, and faster.
Allow us to begin!
Table of Content
10 Things Every Flutter Developer Should Do
1. Make use of constant widgets
Some smart tricks, like using const classes for widgets, can help Flutter apps run faster. This might not seem important, but it is, especially in big apps or when rebuilding views often. Const statements work well with hot-reloading as well, which speeds up the process of making mobile apps. Plus, remember to only use the const phrase when you need to in order to keep things running smoothly. Take a look at this code:
const Container(
width: 100,
child: const Text(‘Hello World’)
);
2. Get rid of some widgets but not the ways
It’s best to keep your code clean and easy to read by breaking your widgets up into smaller parts. But it’s very important to do this right. Some people split items into methods and then call those methods, but that’s not the best way to do it. The problem with this way is that all the widgets inside the methods are rebuilt every time you refresh the main widget that calls all of these smaller widgets (methods). This can hurt performance a great deal. It’s better to separate your widgets into real widgets (classes) to avoid this trouble. This way, you can keep the code easy to read without slowing it down.
3. Whenever you can, stay away from stateful tools.
This tip is especially useful for people who are new to making apps with Flutter. If you can rebuild StatefulWidget and it has all the same benefits as StatelessWidgets, why not use it all the time? StatefulWidget can rebuild itself, but this may slow it down. As a general rule, you should always use StatelessWidget and only use StatefulWidget when you have to. In this way, you can keep your Flutter app creation running smoothly.
4. Take care of the state well
For the best Flutter app speed, state management must work well. Which state management method you choose should depend on how complicated your app is. Most of the time, the built-in setState way is enough for small to medium-sized apps. But for bigger and more complicated apps, it might be a good idea to look into using state management tools like Bloc or Riverpod. These tools can make it easier to handle app states, which will make things run more smoothly and improve performance.
// Bad Approach
setState(() {
// Updating a large data structure unnecessarily
myList.add(newItem);
});
// Better Approach
final myListBloc = BlocProvider.of<MyListBloc>(context);
myListBloc.add(newItem);
5. Make sure your design is clear.
Because Flutter app is a formal framework, it has a less steep learning curve than native frameworks for Android and iOS. It’s easier to learn because it uses the same language for both design and code. But if you don’t set up a clear design, this ease of use can accidentally lead to spaghetti code, which is a mess of conditionally driven code.
This is what we should have at the very least:
To lessen this effect, picking the right design is essential. The pick should be something that the team is familiar with and comfortable with. For example, the Bloc library is a strong choice because it has a lot of well-structured examples that can make code much easier to organize and manage.
6. Follow a good darts style guide
Creating a clear style guide is a good idea because it encourages consistently good writing practices that lead to better code quality. Style consistency not only helps people work together, but it also makes it easier for new Flutter app writers to join the project. For big projects, keeping a regular and uniform style pays off in the long run.
You could make your own style guide that fits the needs of your team, but Dart also has an official style guide that you can use.
It is also strongly suggested that a Linter be added to the project. This tool is especially helpful for bigger teams where not everyone knows the style guide well or might forget to follow some rules from time to time. It makes sure that the quality of the code stays the same.
7. Write tests for important features
When a professional mobile app development business makes Flutter apps for multiple platforms, automated testing saves them a lot of time and work. You can still do manual testing, but because the platform is so big, it’s not possible to test every function carefully after every change.
It would be great if testing covered all of the code, but time and money constraints may make this impossible. Still, it’s important to focus on the most important features and make sure they have enough tests.
Integration tests are important because they let you test on real devices or models.
A helpful tip is to use Firebase Test Lab to run tests on a variety of devices. This will make the app more reliable and compatible.
8. Make the pictures smaller
Improving the performance of your app by optimizing picture files is especially important when working with a lot of images. To keep your computer from running slowly, use picture compression and resizing to make files smaller while keeping the quality you want.
For example, if you have a high-resolution image that you want to use in a smaller app container, don’t use the original high-resolution form. Instead, use a library like flutter_image_compress to quickly change the size of the image to what you want. This helps find a good mix between the quality of the images and how well the app works.
import ‘package:flutter_image_compress/flutter_image_compress.dart’;
// Original image file
var imageFile = File(‘path/to/original/image.png’);
// Get the image data
var imageBytes = await imageFile.readAsBytes();
// Resize and compress the image
var compressedBytes = await FlutterImageCompress.compressWithList(
imageBytes,
minHeight: 200,
minWidth: 200,
quality: 85,
);
// Save the compressed image to a new file
var compressedImageFile = File(‘path/to/compressed/image.png’);
await compressedImageFile.writeAsBytes(compressedBytes);
9. Carefully choose your packages
Packages, which are reuse pieces of code, are very important to the Flutter community because they make the ecosystem more stable. Even though it’s tempting to use Flutter packages for every function, it’s important to think about a few things before adding any package:
Always look to see when the package was last changed. To keep things safe and compatible, don’t use old packages.
Check to see how popular the deal is. It’s usually easier to solve problems with more popular packages because they have more community help.
Check out the package’s code repository to see what problems are still open. Find any problems that haven’t been fixed yet that could affect the features you want to add.
Think about how often changes are sent to the package. When you get changes often, it means that you are using the newest Dart features and fixing bugs.
Some of a package’s features may be more useful than others, so it may be faster to write or copy just that code instead of using the whole package. This cuts down on connections that aren’t needed.
10. Only use streams when you have to
When making mobile apps, streams are a powerful tool, but it’s important to use this power wisely to get the most done. Streams that aren’t set up correctly can use a lot of memory and CPU power, and if you forget to stop them, memory leaks can happen.
Using Streams for a single event may also seem like too much. It is smarter to choose a Future in these situations. Managing various events that happen at different times is best done with streams.
If Streams feels too heavy-handed, you might want to use a lighter option like ChangeNotifier to make responsive user interfaces. This choice could lead to code that is simpler and works better.
Conclusion
Improving the speed of your Flutter app is important for giving users a smooth experience. By using these optimization tips and following best practices for mobile app development, you’re not only making your app faster and smoother, but you’re also building a better user experience that can help your startup stand out in a crowded market.
Of course, if you’re looking for expert teams to make great apps for your business and services, Appic Softwares is a top Flutter app development company with workers who put in the time, effort, and knowledge to make great software solutions.
Remember that if you want to build high-performance Flutter apps, you need to start by thinking about efficiency. By consistently analyzing and carefully coding, you can make apps that look great and work perfectly.
For more details Contact us.