Machine Learning And Property Rate Predection: 101 Guide
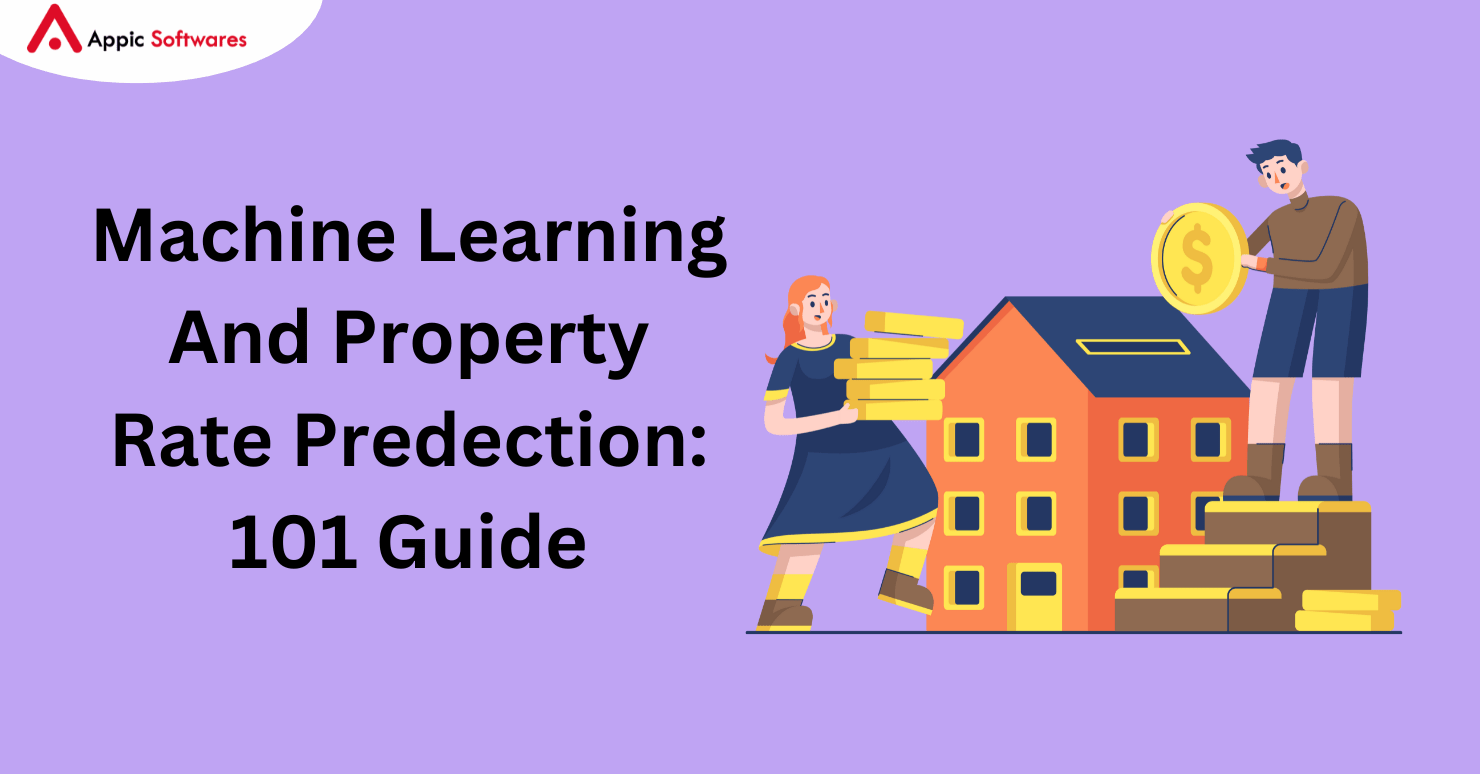
Accurately predicting property prices is challenging in the real estate market since it is a dynamic and constantly shifting landscape, which poses a challenge to buyers, sellers, and investors alike. Algorithms for machine learning (ML) have recently shown great promise in assessing large amounts of data and predicting real estate prices with remarkable accuracy. This paper explores the intriguing field of utilizing ML and Python algorithms to forecast the housing market for the next five years.
ML models are able to understand intricate patterns and relationships that enable them to produce well-informed forecasts about future property prices by utilizing previous property data, including location, size, amenities, and market movements. Because of its extensive library, which includes Scikit-learn, Pandas, and NumPy, Python provides a versatile and easy-to-use framework for creating, training, and implementing these predictive models. In this post, we’ll look at how to use Python and machine learning to predict home values.
Importance and Applications of House Price Prediction
For a number of reasons, house price prediction with Python machine learning is important and has uses in the finance and real estate industries. Accurately predicting home values helps prospective homeowners make wise investment decisions. Setting competitive prices for properties is aided by it for sellers. Better market information and enhanced negotiation tactics are advantageous to real estate brokers.
In order to forecast home values, machine learning algorithms make use of historical property data as well as characteristics like location, square footage, number of bedrooms, and nearby amenities. For this kind of work, sophisticated algorithms like gradient boosting, random forests, and regression are frequently used.
In addition, home price prediction projects are essential for financial planning and risk evaluation for insurers and mortgage lenders. Governments and decision-makers also utilize this data to assess housing market trends and create efficient housing regulations. All things considered, a precise home price forecast project report provides stakeholders with useful information, promoting a more open and effective real estate market.
Importing Libraries and Dataset
Python machine learning for house price prediction entails estimating house prices based on a variety of factors. First, we load the necessary libraries, including Matplotlib, NumPy, Pandas, and Scikit-learn. The property price dataset, which includes details like location, square footage, number of bedrooms, and bathrooms, is then loaded.
# Python Implementation
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
# Load the dataset
data = pd.read_csv(‘house_prices_dataset.csv’)
# Output first few rows of the dataset
print(data.head())
Loading and Preprocessing Data
When utilizing machine learning to anticipate real estate prices, loading and preprocessing data are essential tasks. In order to properly manage the data in this example, Python and popular modules like Pandas and NumPy will be used.
First, the data must be assembled. This data contains details about the house’s location, size, and number of beds. The data is imported using Pandas, a powerful data manipulation tool, which enables us to read the dataset into a data frame.
To ensure the data is clean and suitable for training our model, the following stage is vital data preparation. We apply methods such as Min-Max scaling or standardization to manage missing values, encode categorical variables, and scale numerical characteristics.
An implementation of Python could resemble this:
- python
- import pandas as pd
from sklearn.preprocessing import MinMaxScaler
# Load data
data = pd.read_csv(‘house_prices.csv’)
# Preprocessing
data = data.dropna() # Drop rows with missing values
X = data[[‘HouseSize’, ‘Location’, ‘Bedrooms’]]
y = data[‘Price’]
# Encode categorical variables (if applicable)
# Scale numerical features
scaler = MinMaxScaler()
X_scaled = scaler.fit_transform(X)
# Rest of the machine learning pipeline…
A cleaned and preprocessed dataset that is ready to be used in machine learning models to forecast home values based on input attributes would be the outcome. We may move forward with feature selection, model training, and evaluation for an accurate house price prediction model with this prepared data.
Investigative Data Analysis
Any data analysis project, including the use of machine learning in Python to predict house prices, must include exploratory data analysis (EDA). Prior to developing the prediction model, it entails a process of comprehending, summarizing, and visualizing the primary attributes of the dataset.
For the purpose of predicting house prices, let’s take a look at a dataset that includes attributes like square footage, the number of bedrooms, bathrooms, and locations. Analyzing data distribution, finding missing values, looking for outliers, and investigating correlations between variables are some of the tasks that may be included in the EDA process.
The most widely used Python EDA libraries are Seaborn, Matplotlib, and Pandas. This could be how an example of implementation looks:
Python
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
# Load the dataset
data = pd.read_csv(‘house_data.csv’)
# Data summary
print(data.head())
print(data.info())
print(data.describe())
# Data visualization
plt.figure(figsize=(10, 6))
sns.histplot(data[‘Price’], kde=True)
plt.title(‘House Price Distribution’)
plt.xlabel(‘Price’)
plt.ylabel(‘Frequency’)
plt.show()
plt.figure(figsize=(10, 6))
sns.scatterplot(x=’SquareFootage’, y=’Price’, data=data)
plt.title(‘Price vs. Square Footage’)
plt.show()
# Correlation heatmap
plt.figure(figsize=(10, 8))
correlation_matrix = data.corr()
sns.heatmap(correlation_matrix, annot=True, cmap=’coolwarm’)
plt.title(‘Correlation Heatmap’)
plt.show()
This Python implementation will produce the following results:
- An overview of the data is provided by the dataset’s first few rows.
- Data details include the quantity of non-null items and the kinds of data in each column.
- dataset’s descriptive statistics, such as mean, standard deviation, min, max, and so forth.
Examples of visualizations include heatmaps that highlight the relationships between various features, scatter plots that show the relationship between price and square footage, and histograms that display the distribution of home values.
Data Purification
Building a machine learning model for real estate market predictions requires a critical step: data cleaning. It entails locating and fixing dataset flaws, discrepancies, and missing values.
Python
# Python Implementation
# Handling outliers
data = data[(data[‘price’] >= 100000) & (data[‘price’] <= 1000000)]
# Removing duplicate
data.drop_duplicates(inplace=True)
# Normalizing numerical features
data[‘area’] = (data[‘area’] – data[‘area’].min()) / (data[‘area’].max() – data[‘area’].min())
Data Visualization for Home Price Information
When it comes to deciphering patterns and insights from intricate datasets like home price data, data visualization is essential.
Python
# Python Implementation
# Visualizing the distribution of house prices
plt.hist(data[‘price’], bins=20)
plt.xlabel(‘Price’)
plt.ylabel(‘Frequency’)
plt.show()
# Visualizing the relationship between area and price
plt.scatter(data[‘area’], data[‘price’])
plt.xlabel(‘Area’)
plt.ylabel(‘Price’)
plt.show()
Data Splitting & Feature Selection
Choosing pertinent elements is essential to creating a precise model. After that, we divided the data into testing and training sets.
Python
# Python Implementation
from sklearn.feature_selection import SelectKBest, f_regression
# Feature selection using SelectKBest
selector = SelectKBest(score_func=f_regression, k=5)
X_train_selected = selector.fit_transform(X_train, y_train)
X_test_selected = selector.transform(X_test)
Model Choice and Precision:
This process involves choosing a suitable machine learning algorithm, training it with training data, and assessing how well it performs using test data.
Python
# Python Implementation
from sklearn.ensemble import RandomForestRegressor
from sklearn.metrics import mean_squared_error
# Model training
model = RandomForestRegressor()
model.fit(X_train_selected, y_train)
# Model prediction
y_pred = model.predict(X_test_selected)
# Model evaluation
mse = mean_squared_error(y_test, y_pred)
accuracy = 1 – (mse / np.var(y_test))
print(“Model Accuracy:”, accuracy)
Top Machine Learning and AI Courses Online
Master of Science in Machine Learning & AI from LJMU | Executive Post Graduate Programme in Machine Learning & AI from IIITB | |
Advanced Certificate Programme in Machine Learning & NLP from IIITB | Advanced Certificate Programme in Machine Learning & Deep Learning from IIITB | Executive Post Graduate Program in Data Science & Machine Learning from University of Maryland |
To Explore all our certification courses on AI & ML, kindly visit our page below. | ||
Machine Learning Certification |
Model Assessment
Metrics like accuracy, R-squared, and mean squared error are used to assess the model.
Python
# Python Implementation
from sklearn.metrics import r2_score
# R-squared score
r2 = r2_score(y_test, y_pred)
print(“R-squared:”, r2)
Conclusion
our comprehensive guide on machine learning for property rate prediction equips you with essential knowledge and techniques for accurate real estate valuation. By leveraging advanced algorithms, businesses can make informed decisions and optimize their property investments effectively.
Moreover, if you are looking for a Real estate development company that can help you create a future proof solution then you should check out Appic Softwares. We have an experienced team of Proptech developers that have created several real estate solutions like RoccaBox.
So, what are you waiting for?