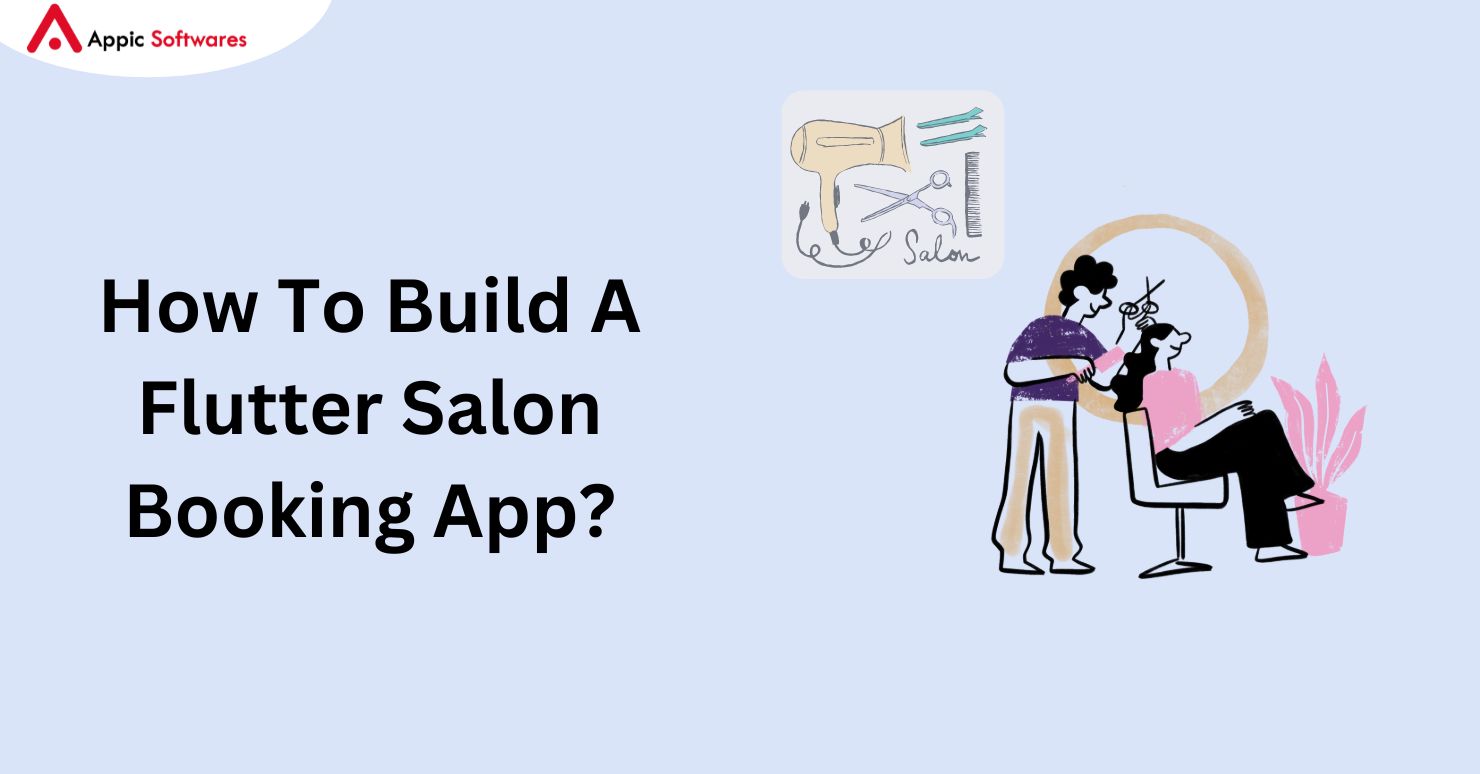
In today’s fast-paced world, the beauty and salon industry is evolving rapidly, with technology playing a significant role in enhancing customer experience and operational efficiency. The size of the world market for salon services was estimated at $215.65 billion in 2022 and is expected to increase to $383.88 billion by 2030 from $230.64 billion in 2023. One of the most impactful developments is the salon booking app, which allows clients to book appointments, browse services, and manage their beauty routines conveniently. Flutter, Google’s UI toolkit, is a powerful tool for developing such apps. This article delves into the process of building a Flutter salon booking app, covering everything from the initial setup to advanced features and deployment.
Why Choose Flutter For Salon Booking Apps?
Cross-Platform Development
Flutter allows you to develop applications for both iOS and Android from a single codebase, significantly reducing development time and costs. This cross-platform capability ensures that your salon booking app reaches a wider audience.
Fast Development And Hot Reload
Flutter’s hot reload feature enables developers to see changes in real time without restarting the application. This accelerates the development process and allows for quicker iterations, leading to faster time-to-market.
Rich UI Components
Flutter provides a rich set of pre-designed widgets and tools, enabling developers to create highly customizable and visually appealing user interfaces. For a salon booking app, this means creating an attractive, user-friendly experience that aligns with your brand.
Strong Community And Support
Flutter has a robust community and extensive documentation, making it easier for developers to find solutions to common issues and stay updated with the latest trends and best practices.
Key Features Of A Salon Booking App
User Registration And Authentication
A seamless registration and authentication process is crucial for user acquisition and retention. Implementing social media login options (e.g., Google, Facebook) can enhance user convenience.
Appointment Booking System
The core feature of a salon booking app is its appointment booking system. Users should be able to select services, choose their preferred stylist, and book appointments based on availability.
Service Catalog
A detailed service catalog with descriptions, pricing, and duration helps users make informed decisions. High-quality images and user reviews can further enhance the catalog.
Real-Time Notifications
Push notifications and in-app notifications keep users informed about their upcoming appointments, special offers, and other important updates.
Payment Integration
Integrating secure payment gateways (e.g., Stripe, PayPal) allows users to pay for services directly through the app. Offering multiple payment options can improve user satisfaction.
Reviews And Ratings
User reviews and ratings for services and stylists provide valuable feedback and help maintain quality standards. They also assist new users in making informed choices.
User Profile Management
Users should be able to manage their profiles, view booking history, and update personal information easily.
Admin Panel
An admin panel allows salon owners to manage appointments, services, staff, and view analytics. This helps in streamlining operations and improving service delivery.
How To Build A Flutter Salon Booking App?
Setting Up Your Flutter Development Environment
Installation
To get started with Flutter, you need to install Flutter SDK and set up your development environment. Follow the official Flutter installation guide for detailed instructions.
Creating A New Project
Once your environment is set up, create a new Flutter project using the following command:
flutter create salon_booking_app
cd salon_booking_app
Choosing Dependencies
Select and install the necessary dependencies for your project. Commonly used packages for a salon booking app might include:
- firebase_auth: For user authentication
- cloud_firestore: For database management
- provider: For state management
- flutter_local_notifications: For sending notifications
- stripe_payment: For payment integration
Building The Core Features
User Registration And Authentication
Implementing user authentication involves setting up Firebase Authentication and integrating it with your app. Allow users to sign up using email/password or social media accounts.
import ‘package:firebase_auth/firebase_auth.dart’;
import ‘package:flutter/material.dart’;
class AuthService {
final FirebaseAuth _auth = FirebaseAuth.instance;
Future<User?> signInWithGoogle() async {
// Implement Google Sign-In
}
Future<User?> registerWithEmail(String email, String password) async {
try {
UserCredential userCredential = await _auth.createUserWithEmailAndPassword(
email: email,
password: password,
);
return userCredential.user;
} catch (e) {
print(e.toString());
return null;
}
}
Future<void> signOut() async {
await _auth.signOut();
}
}
Appointment Booking System
The booking system requires a calendar widget to display available slots and allow users to book appointments. Use TableCalendar or similar packages to implement this feature.
import ‘package:table_calendar/table_calendar.dart’;
import ‘package:flutter/material.dart’;
class BookingScreen extends StatefulWidget {
@override
_BookingScreenState createState() => _BookingScreenState();
}
class _BookingScreenState extends State<BookingScreen> {
CalendarController _calendarController;
@override
void initState() {
super.initState();
_calendarController = CalendarController();
}
@override
void dispose() {
_calendarController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text(‘Book an Appointment’)),
body: Column(
children: <Widget>[
TableCalendar(
calendarController: _calendarController,
onDaySelected: (date, events, holidays) {
// Handle date selection
},
),
// Additional UI for service selection and booking
],
),
);
}
}
Service Catalog
Create a dynamic service catalog using Firestore to store and retrieve service data. Display the services using Flutter’s UI components.
import ‘package:cloud_firestore/cloud_firestore.dart’;
import ‘package:flutter/material.dart’;
class ServiceCatalog extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text(‘Services’)),
body: StreamBuilder(
stream: FirebaseFirestore.instance.collection(‘services’).snapshots(),
builder: (context, snapshot) {
if (!snapshot.hasData) {
return Center(child: CircularProgressIndicator());
}
var services = snapshot.data.docs;
return ListView.builder(
itemCount: services.length,
itemBuilder: (context, index) {
var service = services[index];
return ListTile(
title: Text(service[‘name’]),
subtitle: Text(service[‘description’]),
trailing: Text(‘\$${service[‘price’]}’),
);
},
);
},
),
);
}
}
Real-Time Notifications
Use the flutter_local_notifications package to implement push notifications. Configure Firebase Cloud Messaging (FCM) to send notifications from the server.
Payment Integration
Integrate Stripe or another payment gateway to handle payments securely. Use the stripe_payment package to process payments within the app.
import ‘package:flutter/material.dart’;
import ‘package:stripe_payment/stripe_payment.dart’;
class PaymentService {
PaymentService() {
StripePayment.setOptions(
StripeOptions(
publishableKey: “your_publishable_key”,
merchantId: “your_merchant_id”,
androidPayMode: ‘test’,
),
);
}
Future<void> processPayment(double amount) async {
// Implement payment processing
}
}
Enhancing User Experience
User Reviews And Ratings
Allow users to rate and review services and stylists. Store reviews in Firestore and display them in the app.
User Profile Management
Implement a user profile screen where users can update their information and view their booking history.
import ‘package:flutter/material.dart’;
import ‘package:firebase_auth/firebase_auth.dart’;
class ProfileScreen extends StatelessWidget {
final User user;
ProfileScreen({required this.user});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text(‘Profile’)),
body: Column(
children: <Widget>[
ListTile(
title: Text(‘Name’),
subtitle: Text(user.displayName ?? ‘No name’),
),
ListTile(
title: Text(‘Email’),
subtitle: Text(user.email ?? ‘No email’),
),
// Additional profile information
],
),
);
}
}
Admin Panel
Managing Appointments And Services
Create an admin panel for salon owners to manage appointments, services, and staff. Use a web-based interface with Flutter Web or a separate admin mobile app.
Analytics And Reports
Integrate analytics tools (e.g., Firebase Analytics) to track user behavior and generate reports for business insights.
Deployment And Maintenance
Testing And Debugging
Thoroughly test your app on multiple devices and screen sizes. Use Flutter’s debugging tools to identify and fix issues.
Deployment
Publish your app on the Google Play Store and Apple App Store. Follow the respective guidelines for app submission.
Regular Updates
Keep your app updated with new features and improvements based on user feedback. Regular maintenance ensures a smooth and bug-free experience.
Conclusion
Building a salon booking app with Flutter offers numerous advantages, from cross-platform development to a rich set of UI components. By following this comprehensive guide, you can create a feature-rich and user-friendly salon booking app that meets the needs of both clients and salon owners. Embrace the power of Flutter to streamline salon operations and enhance customer satisfaction, positioning your business for success in the digital age.
Ready to revolutionize your salon business with a cutting-edge booking app? Partner with Appic Softwares, the leading salon app development company, to create a seamless and feature-rich salon booking app using Flutter. With our expertise and commitment to excellence, we can help you streamline your operations, enhance customer satisfaction, and stay ahead in the competitive beauty industry.
Contact us today to get started on your custom salon booking app development journey!