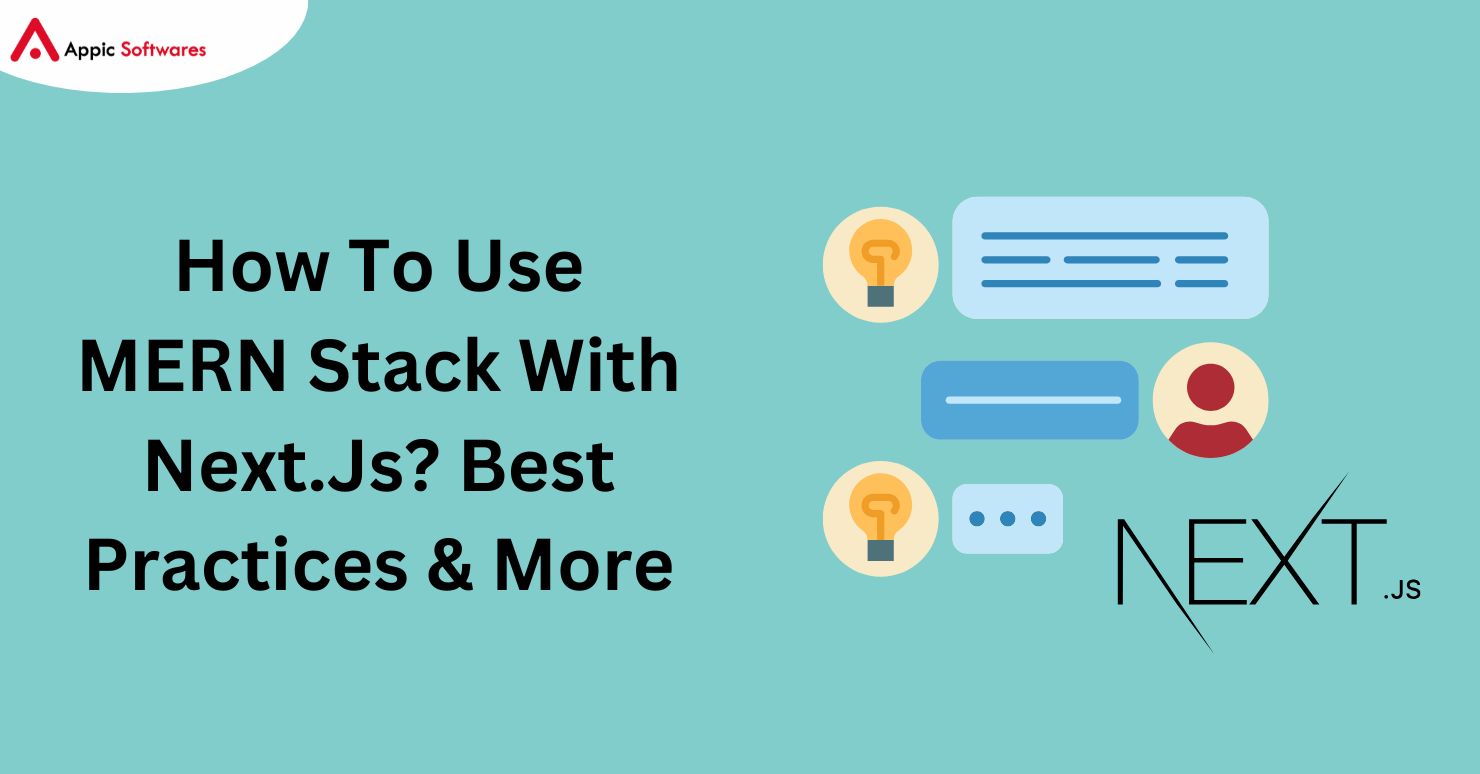
The MERN stack, which consists of MongoDB, Express.js, React, and Node.js, has become a popular choice for developers to build modern web applications. Each of these technologies plays a specific role in creating a full-stack web app: MongoDB serves as the database, Express.js acts as the backend framework, React is responsible for the frontend, and Node.js powers the backend runtime environment. However, when you combine the MERN stack with Next.js, a powerful React-based framework, you unlock additional capabilities such as server-side rendering (SSR), static site generation (SSG), and seamless API routes, making your app more performant and SEO-friendly.
In this detailed guide, we’ll explore how to set up the MERN stack with Next.js, delve into best practices for integrating these two technologies, and provide examples to demonstrate the implementation. Whether you’re looking to build your own app or hire AI engineers to help leverage AI capabilities alongside MERN and Next.js, by the end of this article, you’ll have a solid foundation for building scalable, fast, and optimized web applications.
What Is The MERN Stack?
The MERN stack serves as a full-stack development framework, utilizing JavaScript throughout the entire development process, from frontend to backend. Here’s a breakdown of each component of the stack:
- MongoDB is a NoSQL database that stores data in flexible, JSON-like documents. This allows for easy integration with JavaScript and provides a scalable solution for handling large datasets.
- Express.js is a minimalist web framework for Node.js, designed to handle HTTP requests, routing, middleware, and much more. It is used to create the server-side logic for your application.
- React is a JavaScript library for building user interfaces. It focuses on building reusable components, enabling React web developers to manage the view layer of web applications effectively.
- Node.js is a JavaScript runtime environment that runs server-side code. It allows you to execute JavaScript outside the browser, enabling you to build scalable and fast web servers.
The beauty of the MERN stack is its ability to handle the entire development process with JavaScript, ensuring consistency and a streamlined workflow.
What Is Next.js?
Next.js is a framework built on top of React that allows developers to create highly optimized web applications. It enhances the capabilities of traditional React applications by offering out-of-the-box features like server-side rendering (SSR), static site generation (SSG), and API routes, which improve performance, scalability, and SEO. Next.js also simplifies the process of creating dynamic pages and integrating backend logic directly into the project, all while maintaining React’s component-based structure.
Why Use MERN Stack With Next.js?
The combination of the MERN stack with Next.js allows developers to build full-stack web applications that are performant and scalable and provide enhanced user experiences. Here are some of the key reasons to integrate these two technologies:
- Improved Performance: Pages built with static site generation (SSG), like in Next.js, can improve load times by up to 50%, which is crucial as 53% of mobile users abandon websites that take more than 3 seconds to load. With Next.js, you can choose between static site generation (SSG) and server-side rendering (SSR), depending on the type of content you’re serving. Static pages are pre-built at compile time, allowing them to load faster. For dynamic content, SSR provides a seamless way to serve updated data without sacrificing speed.
- Simplified Routing: Next.js comes with built-in routing, eliminating the need for third-party libraries like React Router. Pages are created by simply adding a file to the /pages directory, and routes are automatically generated based on the file structure.
- Enhanced Flexibility: The combination of Node.js on the backend and Next.js on the frontend provides a flexible development environment where you can build complex web applications. You can also integrate API routes directly into your Next.js application, reducing the need for a separate server setup.
- Full-stack JavaScript: From interacting with databases to rendering on the front end, the whole development process is done in JavaScript. This makes the workflow consistent and fast for developers who know the language.
Read more: Difference Between Page Router Vs App Router In Next.Js
Setting Up The MERN Stack With Next.js
To get started with building a MERN stack application using Next.js, the first step is to set up the environment by installing the necessary dependencies, structuring the project, and configuring the backend.
1. Installing Dependencies
Begin by installing the essential tools and libraries for both the MERN stack and Next.js. Here’s a step-by-step guide on how to do this:
- Create a Next.js project: Open your terminal and run the following command to create a new Next.js project:
- Install backend dependencies: Since Next.js handles the frontend, you’ll need to install Express.js for the backend and Mongoose to interact with MongoDB:
- Install frontend dependencies: By default, React and React-DOM come installed with Next.js, so no additional installation is required for the frontend.
2. Backend Configuration With Node.js And Express
To set up the backend server, create a server.js file in the /backend directory. This file will handle the server-side logic for your app, such as setting up routes and connecting to MongoDB.
Here’s an example of a basic Express.js server:
In your .env file, you will store sensitive information like MongoDB connection strings. Here’s an example:
3. Integrating MongoDB
Now that the backend server is up and running, you can create models to define the structure of the data stored in MongoDB. For example, to create a simple model for users, you would add the following:
Using Next.js With The MERN Stack
Once the backend is configured, you can begin integrating Next.js with the MERN stack. One of the biggest advantages of Next.js is its ability to offer multiple rendering methods, including Server-Side Rendering (SSR), Static Site Generation (SSG), and Client-Side Rendering (CSR).
Server-Side Rendering (SSR)
Server-Side Rendering allows you to render pages dynamically on the server at the time of the request. This can improve SEO and performance for dynamic content. To use SSR in Next.js, you need to use getServerSideProps:
Static Site Generation (SSG)
For static content that doesn’t change frequently, you can use Static Site Generation. This builds static HTML files during the build process, which can drastically improve page load times.
Best Practices For MERN Stack With Next.js
Code Splitting
It is built into Next.js. This means that it only loads the JavaScript that is needed for the present page. This speeds things up by cutting down on the code that needs to be loaded at startup. This is especially helpful for big apps with lots of parts. Code splitting makes sure that users only download the code they need for the page they are looking at, so the whole app doesn’t load at once, something that Next.Js development companies often leverage to build fast and efficient applications.
API Routes
Building-in API routes are one of the best things about Next.js. With these routes, you can describe backend logic in the same app, so you don’t have to set up a separate server. It’s easy to handle and organize both your frontend code and the backend functionality because each API route is linked to a file in the /pages/api directory. To enhance security, many developers integrate Auth0 Next.Js Authentication for seamless and secure user authentication within these API routes.
Optimized Images
Image optimization is an important part of web performance, and Next.js has an answer for it right out of the box. It also allows lazy loading, which means that images aren’t loaded until they reach the viewport. This speeds up the initial page load time and saves users bandwidth.
State Management
The state of different parts of your application can get hard to manage as it grows. You can use the Context API from React or a state management library like Redux with Next.js to control the overall state of your app. The Context API makes it easy to pass state from one component to another in the tree of components without having to pass props by hand at each level.
Conclusion
Integrating the MERN stack with Next.js can significantly enhance your web development process. By utilizing the strengths of both technologies—MongoDB for a powerful NoSQL database, Express.js for robust server-side logic, React for modern frontend development, and Next.js for optimized rendering—you can build scalable, SEO-friendly, and performant web applications. Whether you’re working on a simple project or a complex, data-driven application, this combination provides the tools necessary to succeed.
If you’re ready to streamline your development process and harness the best of modern web tech, this guide is the perfect place to start.
Want to build faster, smarter apps? Partner with Appic Softwares, let’s get your MERN and Next.js project rolling today!