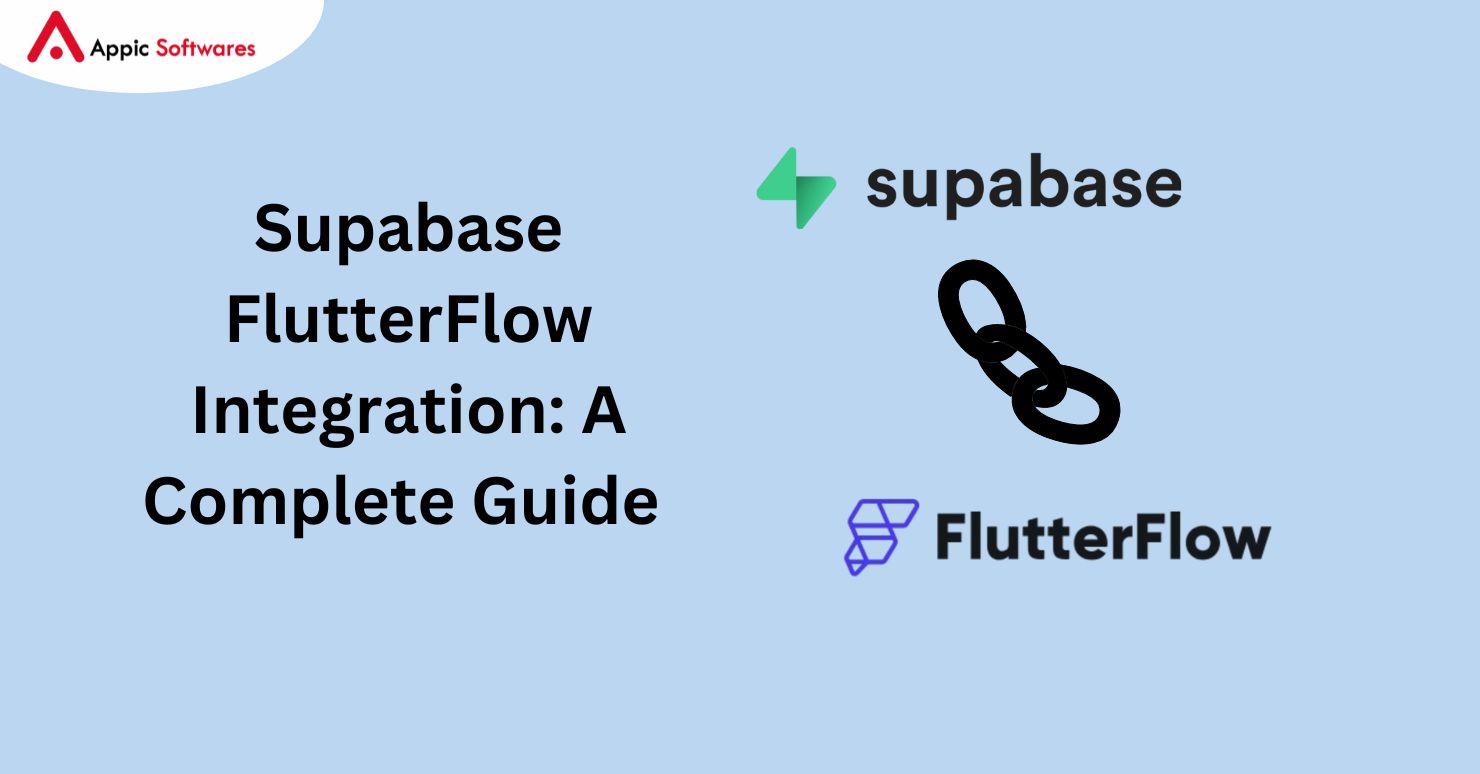
Integrating Supabase with FlutterFlow offers a seamless way to enhance your app development process, combining the backend-as-a-service capabilities of Supabase with the powerful UI-building features of FlutterFlow. This guide provides a comprehensive walkthrough for setting up and integrating Supabase in your FlutterFlow projects, ensuring efficient and scalable app development.
What Is Supabase?
Supabase is an open-source Firebase alternative that offers a suite of backend services like authentication, real-time databases, and storage. FlutterFlow, on the other hand, is a low-code platform for building cross-platform Flutter applications. By integrating these two powerful tools, developers can create robust, real-time applications with minimal effort.
Setting Up Supabase In Flutter
To start integrating Supabase into your Flutter project, you first need to initialize a new Flutter application. Open your terminal and run the following command:
flutter create your_project_name
This command sets up the basic structure for your Flutter project.
Installing Dependencies
Next, add the supabase_flutter package to your pubspec.yaml file. This package includes all the necessary functionalities to work with Supabase in your Flutter app.
dependencies:
flutter:
sdk: flutter
supabase_flutter:
Source: Restack
After adding the dependency, install it by running:
flutter pub get
Configuring Deep Links
Deep links are essential for handling authentication callbacks, especially when using magic link authentication. Configure deep link settings in both your Flutter project and the Supabase dashboard.
In Flutter Project
For iOS, open the Info.plist file and add the following:
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleURLSchemes</key>
<array>
<string>io.supabase.flutterquickstart</string>
</array>
</dict>
</array>
For Android, open the AndroidManifest.xml file and add the following:
<intent-filter>
<action android:name=”android.intent.action.VIEW”/>
<category android:name=”android.intent.category.DEFAULT”/>
<category android:name=”android.intent.category.BROWSABLE”/>
<data android:scheme=”io.supabase.flutterquickstart”/>
</intent-filter>
Source: Restack
In Supabase Dashboard
Navigate to the Authentication settings in the Supabase dashboard and set the deep link URL to match your Flutter project’s configuration:
io.supabase.flutterquickstart://login-callback/
Initializing Supabase Client
In your main.dart file, initialize the Supabase client with your project’s URL and anon key. This step is crucial for connecting your Flutter app to your Supabase project.
import ‘package:flutter/material.dart’;
import ‘package:supabase_flutter/supabase_flutter.dart’;
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Supabase.initialize(
url: ‘YOUR_SUPABASE_URL’,
anonKey: ‘YOUR_SUPABASE_ANON_KEY’,
);
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text(‘Supabase FlutterFlow Integration’),
),
body: Center(
child: Text(‘Hello, Supabase!’),
),
),
);
}
}
Source: Restack
Implementing User Authentication
Supabase offers various authentication methods, including email/password, OAuth, and magic links.
Email Authentication
For email authentication, you can use the SupaEmailAuth widget, which provides a seamless UI for sign-in, sign-up, and password recovery.
import ‘package:supabase_flutter/supabase_flutter.dart’;
class EmailAuth extends StatelessWidget {
@override
Widget build(BuildContext context) {
return SupaEmailAuth(
onSuccess: (response) {
// Handle successful authentication
},
onError: (error) {
// Handle authentication errors
},
);
}
}
Source: Restack
Magic Link Authentication
Magic link authentication can be implemented using the SupaMagicAuth widget, allowing users to sign in with a link sent to their email.
import ‘package:supabase_flutter/supabase_flutter.dart’;
class MagicAuth extends StatelessWidget {
@override
Widget build(BuildContext context) {
return SupaMagicAuth(
onSuccess: (response) {
// Handle successful authentication
},
onError: (error) {
// Handle authentication errors
},
);
}
}
Source: Restack
OAuth Authentication
For OAuth authentication, such as signing in with Google or Apple, configure the OAuth providers in the Supabase dashboard. Then, use the appropriate methods in your Flutter app:
import ‘package:supabase_flutter/supabase_flutter.dart’;
class OAuthAuth extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ElevatedButton(
impressed: () async {
final response = await Supabase.instance.client.auth.signInWithProvider(Provider.google);
if (response.error == null) {
// Handle successful authentication
} else {
// Handle authentication errors
}
},
child: Text(‘Sign in with Google’),
);
}
}
Source: Restack
Database Interaction
To interact with the Supabase database, use the Supabase client to perform CRUD operations on your database tables.
Fetch Data
final response = await Supabase.instance.client
.from(‘your_table’)
.select()
.execute();
if (response.error == null) {
final data = response.data;
// Process the data
}
Insert Data
final response = await Supabase.instance.client
.from(‘your_table’)
.insert({‘column’: ‘value’})
.execute();
if (response.error == null) {
// Data inserted successfully
}
Update Data
final response = await Supabase.instance.client
.from(‘your_table’)
.update({‘column’: ‘new_value’})
.eq(‘id’, ‘your_id’)
.execute();
if (response.error == null) {
// Data updated successfully
}
Delete Data
final response = await Supabase.instance.client
.from(‘your_table’)
.delete()
.eq(‘id’, ‘your_id’)
.execute();
if (response.error == null) {
// Data deleted successfully
}
Real-time Updates
Supabase’s real-time capabilities allow you to subscribe to changes in your database, keeping your app’s data up to date.
final subscription = Supabase.instance.client
.from(‘your_table’)
.on(SupabaseEventTypes.insert, (payload){
// Handle insert events
})
.on(SupabaseEventTypes.update, (payload) {
// Handle update events
})
.on(SupabaseEventTypes.delete, (payload) {
// Handle delete events
})
.subscribe();
Source: Restack
Launching the Flutter App
To launch your Flutter app, use the Flutter Run command in your terminal. This command compiles the Dart code into native code and starts the app on a connected device or emulator.
flutter run
For web applications, you can specify the web server settings:
flutter run -d web-server –web-hostname localhost –web-port 3000
Navigate to http://localhost:3000 in your browser to see the app running.
Tips for a Smooth Launch
- Ensure all dependencies are installed by running Flutter pub get.
- Check that your device or emulator is connected and recognized by Flutter.
- For the web, make sure no other services are running on the same port.
Troubleshooting Common Issues
Dependency Conflicts
- Ensure that the supabase_flutter package version is compatible with other packages in your pubspec.yaml.
- Run Flutter pub upgrade to get the latest compatible versions.
Authentication Errors
- Verify the correct setup of OAuth providers in the Supabase dashboard.
- Check for correct usage of signInWithProvider methods for Google or Apple sign-in.
Database Connection
- Confirm that the database URL and anon key are correctly set in Supabase. initialize.
- Use Row Level Security policies to protect your data while allowing necessary access.
Deep Link Configuration
- Ensure that the deep link scheme and host are correctly configured in both the Supabase dashboard and your app’s platform-specific settings.
Performance Issues
- Optimize queries by using indexes and avoiding unnecessary data fetching.
- Consider using the edge_http_client for improved performance in edge environments.
Conclusion
Integrating Supabase with FlutterFlow enables developers to build powerful, real-time applications with ease. By following this guide, you can set up and configure Supabase in your Flutter projects, implement robust user authentication, interact with your database, and handle real-time updates seamlessly. This integration not only simplifies backend management but also enhances the overall development experience, allowing you to focus more on building amazing user experiences.
Ready to take your Flutter app to the next level? Integrate Supabase with FlutterFlow for a seamless, powerful, and scalable backend solution. Follow our comprehensive guide to get started and ensure your app is equipped with real-time capabilities, robust authentication, and efficient database interactions.
For expert assistance and top-notch development services, look no further than Appic Softwares. As the leading FlutterFlow development company, Appic Softwares specializes in creating high-quality, dynamic applications that meet your business needs. Contact us today to get started on your next project and transform your app development process!
FAQs
Q. What is Supabase, and how does it work with FlutterFlow?
Supabase is an open-source backend as a service (BaaS) that offers features like database, authentication, storage, and real-time data. When combined with FlutterFlow, it allows you to build powerful, scalable apps with little to no code.
Q. What kind of apps can I build using Supabase and FlutterFlow together?
You can build a wide range of apps like social media platforms, chat apps, CRMs, eCommerce apps, or anything that requires user authentication, databases, and real-time updates.
Q. What’s the best way to learn Supabase integration in FlutterFlow?
Start with official documentation, video tutorials, or step-by-step guides like this one. You can also explore community forums or reach out to a FlutterFlow development company for expert support.