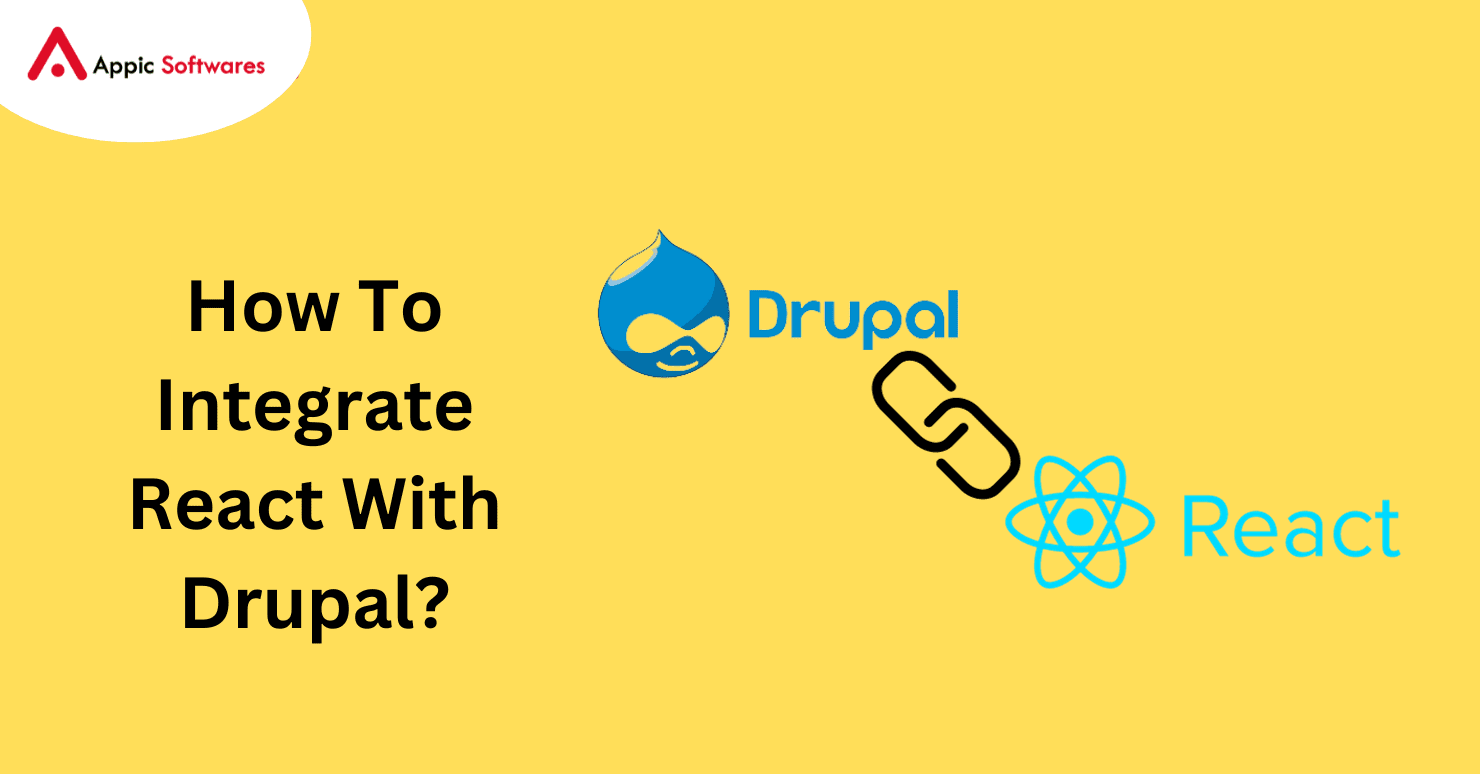
Before we delve into the steps of integrating React with Drupal, it’s important to understand the benefits of this combination. React is a versatile, component-based JavaScript library that excels at building intricate, high-quality front-end interfaces. Drupal, on the other hand, is a robust content management framework known for its flexibility and ability to create content-rich web experiences. By merging React’s exceptional front-end theming capabilities with Drupal’s powerful back-end infrastructure, you can craft remarkable and fast-loading digital experiences. Now, let’s take a closer look at developing React components in Drupal 9.
Understanding React
React is a declarative, effective, and flexible JavaScript library for developing front-end user interfaces, according to its definition. It enables you to build intricate user interfaces out of discrete, small pieces of code known as components.
Understanding the fundamental components of React should be simple for you if you are familiar with Pattern Lab. Starting with the fundamental components or “atoms” of a page, we develop designs from the bottom up.
The Role of JSX In React
The syntax and coding style of React can get complicated and are not very developer-friendly. This is how an HTML element is made in React, as you can see in the example below.
const element = React.createElement (
‘h1’,
{className: ‘greeting’},
‘Hello, world!’
);
Source: Specbee
And using JSX, this is how you would create the same method in React:
const element = <h1 className=”greeting”>Hello World!</h1>
Source: Specbee
The name of the class can be specified using JSX using the preset “className” XML syntax, as seen below. As you can see, writing the same code in JSX is more simpler, more natural, and less verbose. As a result, practically the entire React community writes React code using JSX.
JSX: What is it? JavaScript XML is referred to as JSX. For developers, XML has a very user-friendly syntax. React’s use of JSX makes adding and writing HTML simpler.
Examine the differences between building elements in Javascript and React. You’ll observe that React allows you more freedom when writing and accessing code, which facilitates development and debugging.
React | Javascript |
const Button = <button onClick={console.log(‘clicked!’)}>Click Me</button> |
HTML FILE<button id=”my-button”>Click Me</button> JS FILEfunction onClick() { |
Although JSX makes it simple for developers to write code, browsers have trouble understanding it. Because of this, we will compile it into a standard JavaScript format and provide it to the browser.
Managing Data With React Forms
There are two types of React data forms:
- Props: Properties are gifts from parents and grandparents in the component hierarchy. They are immutable and cannot be altered. You could use a prop to, for instance, send a variable from a parent component to a child component.
- State: A component’s local state can be altered by occurrences. For instance, the text of a state variable (state.text) may vary in response to certain activities.
Note: Through props, child components can get both the state’s values and events for updating it.
Integrating React with Drupal: A Step-by-Step Guide
The JSX file will be created after we first create a custom module.
Let’s now construct the custom Drupal module as demonstrated below:
Create modules/react/react.info.yml
web > modules > react > ! react.info.yml
1 name: React
2 type: module
3 description: ‘A module for basic React components.’
4 core_version_requirement: *8.7.7 || ^9
Source: Specbee
Let’s create the React file as it is depicted below next. The react-app id will be printed in the HTML markup that we are producing with an H1 tag.
Create modules/react/js/src/index.jsx
web > modules > react > js > src > # index.jsx
1 import React from ‘react’;
2 import ReactDOM from ‘react-dom’;
3
4 ReactDOM. render(
5 <h1>Hello there – world!</h1>,
6 document. getElementById( ‘ react—app’)
7 );
Source: Specbee
Accessing JSX Files In React
You must now configure a JavaScript toolchain using Webpack in order for Drupal to read and utilize the JSX file that we just built.
In a nutshell, we’re setting up a procedure that will take our source JavaScript files, such as index.jsx, and run them through several build phases, producing a single, optimized.js file in the end. While developing our Drupal module or theme, we may utilize the entire React/JavaScript ecosystem by using this build step.
Basic actions include:
Create a toolchain that will convert your JavaScript resources into one or more “bundled” JavaScript files with a fixed location.
In order to use the bundled assets from your development toolchain, create a Drupal asset library.
Step 1: Install React, Webpack, and Babel
You must make sure that node and nmp are prepared in your development environment before installing these. The Javascript library is called React. You need the compilers Babel and Webpack to change the.jsx file format into javascript for the browser. One of the greatest community compilers for.jsx files is Babel. Run the scripts below in your terminal from the root directory of your theme’s modules/react/ to install React, Webpack, and Babel.
# Create a package.json if you don’t have one already.
2 npm init -y
3 # Install the required dependencies
4 npm install —-save react react-dom prop—types
5 npm install —-save-dev @babel/core @babel/preset-env @babel/preset-react babel-loader webpack webpack-cli
Step 2: Configure Webpack with a webpack.config.js file
Make a webpack.config.js file and place it in modules/react/webpack.config.js at the module’s root.
1 const path = require(‘path’);
3 const config = {
4 entry: {
5 main: [“./js/src/index.jsx”]
6 },
7 devtool:’source-map’,
8 mode:’development’,
9 output: {
10 path: path.resolve(__dirname, “js/dist”),
11 filename: ‘[name].min.js’
12 },
13 resolve: {
14 extensions: [‘.js’, ‘.jsx’],
15 },
16 module: {
17 rules: [
18 {
19 test: /\.jsx?$/,
20 loader: ‘babel-loader’,
21 exclude: /node_modules/,
22 include: path.join(__dirname, ‘js/src’),
23 }
24 ],
25 },
26 };
27 module.exports = config;
Source: Specbee
Step 3: Configure Babel with a .babelrc file
Create a.babelrc file in the module’s root directory with the following easily accessible presets (which will be used when generating the React code) to provide Babel with some configuration: modules/react/.babelrc
web > modules > react > .babelrc >…
1 {
2 “presets”: [
3 “@babel/preset-env”,
4 “@babel/preset-react”
5 ]
6 }
Source: Specbee
Step 4: Add some helper scripts to package.json
Since your nmp package always references to the base file package, you must do this in order to launch it.Before taking any action, json.
> Debug
“scripts”: {
“build”: “webpack”,
“build:dev”: “webpack”,
“start”: “webpack —-watch”
},
Source: Specbee
Step 5: Run build to compile the JSX files to the single js file
Now we are ready to compile the JSX file.
npm run build (OR) npm run build:dev
In essence, this compiles the file according to the configuration in the webpack.config file, and it then builds the compiled js file and uploads it to the specified repository, js/src/dist.
Step 6: Define a Drupal asset library
The built JavaScript file can now be added to the Drupal library (libraries.yml) as an asset.
web > modules > react > ! react.libraries.yml
1 react_app:
2 version: 1.0
3 js:
4 js/dist/main.min.js: {minified: true}
Source: Specbee
Now that your Drupal website is complete, you can start building some beautiful React components.
Conclusion
So, what do thing, was it easy to integrate React with Drupal? Make sure to build the React dom element in Drupal (‘react-app’) while developing React components, and always add the Javascript file (which we generated from the React JSX file) to the Drupal library.
However, if you are looking for a Drupal development company that holds their expertise in React development as well, then you should check out Appic Softwares. We have an experienced team of Drupal developers that can help you generate more revenue from your store.
So, what are you waiting for?
This blog is inspired by <https://www.specbee.com/blogs/how-to-react-quickly-in-drupal>